Beyond Identity + NextAuth.js
.jpg)
What is NextAuth.js?
If you are implementing authentication in Javascript, then chances are you’ve heard of NextAuth.js (becoming Auth.js). Auth.js is a complete open-source authentication solution for web applications. It is designed from the ground up to support Next.js and Serverless.
It provides a simple and easy-to-use interface for implementing various authentication providers such as Google, Facebook, Twitter, and many more. It is an open-source library that is built on top of OAuth 2.0 and OpenID Connect (OIDC) protocols, making it secure and widely compatible with a variety of services.
Auth.js abstracts away the complexities of authentication and provides a unified API to work with different authentication providers. It simplifies the process of setting up authentication in your Next.js application and allows you to focus on building the core features of your application.
Their repository can be found here: https://github.com/nextauthjs/next-auth
What is Beyond Identity?
Beyond Identity is a company whose mission is to empower all people and businesses to securely, privately, and effortlessly control their digital identities. We provide you, the developer, with passkey-based sign on for your app.
There are no more passwords, even usernames become optional, and the entire experience is better and more secure for your users.
For more information, check out our blog covering our product for developers.
Auth.js + Beyond Identity
We are proud to announce that Beyond Identity is an official supporter and proud contributor of Auth.js. We are happy to introduce our Beyond Identity Provider, which as of Release @auth/core@0.5.0 allows developers to integrate our passwordless experience, along with our JavaScript Embedded SDK or Hosted Web Authenticator.
We have also released a new NextAuth Integration Guide.
Example
TL;DR: Here are some sample code snippets to help you get started!
In this post, we will cover the basics of Auth.js, including how to set it up and use it in your Next.js application.
Beyond Identity developer account
Before we get started, let’s sign up for your free Beyond Identity developer account.
You can read all about how to configure your developer account in out https://developer.beyondidentity.com/docs/v1/workflows/account-setup guide, but for this example, we will need three things:
- A Realm, which is a namespace in your tenant that isolates identities and applications from other realms.
Give it a Name! For the purposes of this example, we will call it Example.

- An Application, which is an abstraction in the admin console that enables you to configure passkey binding and authentication in your mobile/web apps.
Give it a Display Name! For the purposes of this example, we will call it Example.
The important things to note here are Client Type, which should be Confidential, and PKCE, which should be S256.
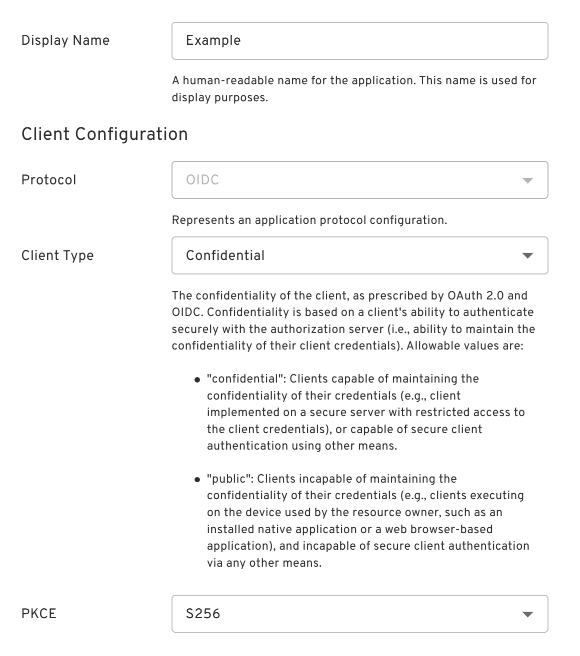
- An Authenticator Config, which is used to tell us how and where to invoke passkey creation and authentication flows for your native/mobile or web application.
The important thing to note here is Configuration Type. This can be either Hosted Web or Embedded SDK, with an Invocation Type of Automatic, but for the purposes of this example, we will choose Hosted Web.
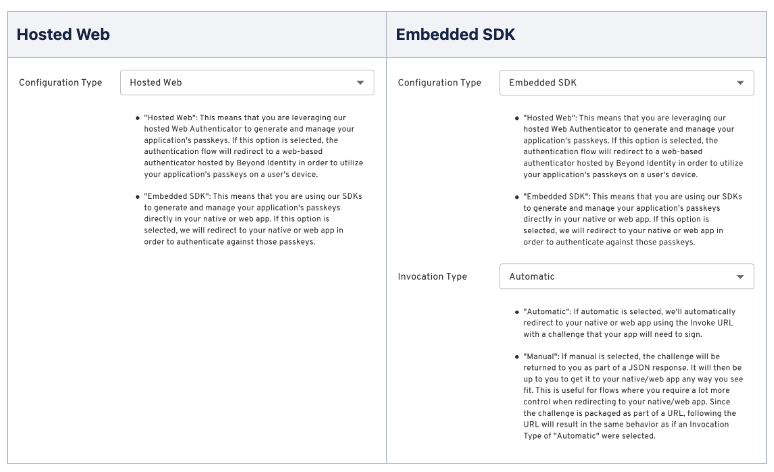
Installing Auth.js
To get started with Auth.js, you need to install it in your Next.js application. You can do this by running the following command in your terminal:
npm install --save @auth/core
Alternatively, if you are using Yarn, you can run the following command:
yarn add --save @auth/core
Once the installation is complete, you can import Auth.js in your application:
import { Auth } from "@auth/core"
Configuring Auth.js
Next, you need to configure Auth.js by creating a pages/api/auth/[...nextauth].js
file. This file will handle authentication requests and provide authentication callbacks to your application. Here is an example configuration for Auth.js that supports authentication with Beyond Identity:
import { Auth } from "@auth/core"
import BeyondIdentity from "@auth/core/providers/beyondidentity"
const request = new Request("https://example.com")
const response = await Auth(request, {
providers: [
BeyondIdentity({
clientId: process.env.BEYOND_IDENTITY_CLIENT_ID,
clientSecret: process.env.BEYOND_IDENTITY_CLIENT_SECRET,
issuer: process.env.BEYOND_IDENTITY_ISSUER,
}),
],
})
In this example, we are configuring Auth.js to use the Beyond Identity authentication provider. We pass in the issuer
, clientId
and clientSecret
that we obtained from the Beyond Identity Admin Console you created in the first step.
You can add additional authentication providers by including them in the providers
array.
Conclusion
Feel free to reach out on Slack if you have any questions! Happy coding!
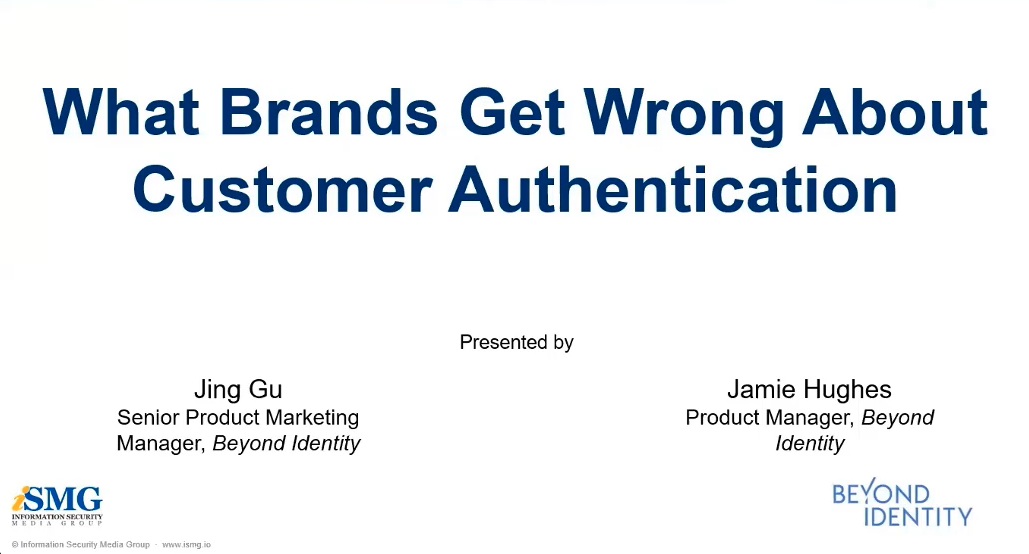